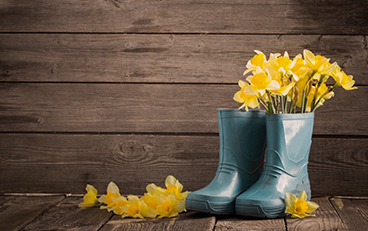
Why Migrate Spring Boot Versions?
Spring Boot is an open source Java-based framework used to create microservices. Developed and maintained by Pivotal (now VMWare Tanzu), it is used to build stand-alone and production ready Spring applications. With annotation configuration and default codes, Spring Boot shortens the time involved in developing an application. It helps create a stand-alone application with less or almost zero-configuration. Auto configuration is a special feature of Spring Boot. It automatically configures a class based on that requirement.
Spring Boot 2.x has been released and is available now from repo.spring.io, Maven Central and Bintray. This release adds a significant number of new features and improvements. Applications will now start even faster and consume less memory. It can be particularly beneficial in environments with very tight memory constraints. Spring Boot 2.2 also supports Java 13, while remaining compatible with Java 11 and 8.
Migration Initiation Steps
Add the migrator dependency into the pom.xml file. This should be removed after finishing Spring Boot Migration.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-properties-migrator</artifactId>
<scope>compile</scope>
</dependency>
Run the migration in debug mode to see everything that is occurring
logging.level:
org.springframework.web: DEBUG
com.fasterxml.jackson: DEBUG
org.hibernate: DEBUG
Migrate Dependencies
As shown below, some dependencies (in our case, we are using keycloak for example) may need to be upgraded to be compatible.
keycloak dependencies: minimum required for spring boot 2.x is 4.4.0.Final.
<dependency>
<groupId>org.keycloak</groupId>
<artifactId>keycloak-spring-boot-starter</artifactId>
<version>4.4.0.Final</version>
</dependency>
<dependency>
<groupId>org.keycloak</groupId>
<artifactId>keycloak-spring-security-adapter</artifactId>
<version>4.4.0.Final</version>
</dependency>
org.springframework.data dependencies: minimum required for Spring boot 2.x is 2.0.0.RELEASE.
org.powermock dependencies: upgrade into mockito2.
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-api-mockito2</artifactId>
<version>1.7.1</version>
<scope>test</scope>
</dependency>
In case you get the following error:
java.lang.NoSuchMethodError: org.mockito.internal.handler.MockHandlerFactory.createMockHandler(Lorg/mockito/mock/MockCreationSettings;)Lorg/mockito/internal/InternalMockHandler;
you need to upgrade the appropriate package (e.g. mockito version)
<powermock.version>2.0.0-beta.5</powermock.version>
Custom Configuration
- When we update Spring Boot to the 2.2.5.RELEASE, we need to add the following into the properties:
<maven-jar-plugin.version>3.1.1</maven-jar-plugin.version>
- Due to actuator vulnerability issues with Spring Boot 1.x., a custom configuration needs to be implemented in the “application.yml” file. After upgrading to Spring Boot 2.x, the following properties should be deleted:
#Endpoint sensitive flag is no longer customizable as Spring Boot no longer provides a customizable security auto-configuration
endpoints:
#/info endpoint configuration
info:
id: info
sensitive: false
enabled: true
#/metrics endpoint configuration
metrics:
id: metrics
sensitive: false
enabled: true
#/health endpoint configuration (Comment when you are using customized health check)
health:
id: health
sensitive: false
enabled: true
#The security auto-configuration is no longer customizable.
#securing endpoints by spring security
#security:
# basic:
# enabled: false
remove version from spring-web dependency
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.3.10.RELEASE</version>
</dependency>
NEW JPA API For Spring Boot 2.X
CrudRepository changed its name of methods and also started to return Optional type by default.
The methods changed from operation on collections:
delete() -> deleteAll() save() -> saveAll()
And, also with findById now returns an Optional:
findOne() -> findById()
findById() returns an Optional now, so it’s a good idea to use orElseThrow() and throw an exception if the element was not found.
Possible Errors and Solutions
Some errors may occur when running the Spring Boot 2.x application. Below are some of these errors and fixes.
- Error processing condition on “org.springframework.cloud.autoconfigure” solution:
Solution: remove version from spring-web dependency
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.3.10.RELEASE</version>
</dependency>
- com.amazonaws.SdkClientException: Failed to connect to service endpoint:
at com.amazonaws.internal.EC2ResourceFetcher.doReadResource(EC2ResourceFetcher.java:100) [aws-java-sdk-core-1.11.745.jar:na]
Solution: correct version is:
<aws.version>1.11.656</aws.version>
- To fix the error org.postgresql.jdbc.PgConnection.createClob() is not yet implemented
Solution: the following changes should be added to the properties file
spring.jpa.properties.hibernate.jdbc.lob.non_contextual_creation=true
Conclusion
A key element of Spring Boot is infrastructural support at the application level. It focuses on the “plumbing” of enterprise applications, so that teams can focus on application-level business logic without unnecessary ties to specific deployment environments. Spring Boot is the optimal choice for one of the microservices application projects for our client. It creates all the application services and manages the communication between them, enabling developers working with this framework to involve different technologies.
Upgrading from Spring Boot 1.x to 2.x involves more work than what listed in the steps above. It is not surprising considering that it’s not only a migration of Spring Boot, but also of Spring, Hibernate and many more libraries.